The Oxford Dictionary defines an algorithm as a process or set of rules to be followed in calculations or other problem-solving operations, especially by a computer.
You may not need an algorithm when creating a web page. But say, you need to create a program that does a word search or a program that forecasts sales, what logic will give the optimum solution? An algorithm outlines the logical steps to take to get an optimum solution.
Let’s swap algorithm
Say, you have a bucket of apples and a bucket of oranges. You need to swap them.
Approach 1: Empty all the apples into the bucket of oranges. Pick up each orange and drop them into the empty bucket. This isn’t very efficient because it takes time to fish out the oranges. This will not work if you have to swap, say, two buckets of sand!
Approach 2: Better approach is to get a third bucket. Pour all contents of bucket 1 into bucket 3. Pour bucket 2 into bucket 1 and bucket 3 into bucket 2. They are now swapped. It’s a much quicker approach and works for any swapping!
Algorithms don’t need a programming language. It’s written in plain English or a mix of plain English and simple math statements. Once you have an algorithm, you can develop the actual program using a programming language.
An algorithm has a defined input, the detailed sequence of steps to be processed by the computer and the expected output.

An ordered list of steps written in plain English, that takes an input and produces an output is called an Algorithm.
An algorithm has specific set of instructions. Write out each step in sequence and specify how each input is processed and what comes next till you arrive at the final output. Be detailed in each step, so there is no ambiguity. A computer relies on instructions it receives. Bad input results in bad output.
Algorithm to calculate the Average.
- Read input numbers.
- Count the number of numbers to give Count_of_Numbers.
- Add all the numbers to give you Total.
- Divide Total by Count_of_Numbers to give the Average.
Can you write an algorithm to find if two words are anagrams? Anagrams use the same set of letters to form two words like ‘rate’ and ‘tear’.
Difference between an Algorithm and a Program
While an algorithm is written as a sequence of steps in plain English that serves as a way to step through a solution, it is not executable by a computer. You need to write a program using a programming language using the same logic that can be executed by a computer. We will learn more about programming in the next topic
ALGORITHM ACTIVITY
Write an algorithm for making lemonade.
Below is what I have. Do not look at it yet and come up with your own steps. Once done, you may look at below. How does yours compare? Is something missing from below? Remember, there is no wrong answer. The more details you have, the better you will be able to cover all possibilities.
..
..
..
1. Get a container.
2. Get ingredients – 2 lemons, 1/2 cup sugar, 2 cups of water, ice, a pinch of salt.
3. Pour water into the container.
4. Add the sugar and pinch of salt.
5. Squeeze lemon juice into the container.
6. Stir for 2 minutes.
e. Add ice.
Done. Lemonade is ready.
Pseudocode – Pseudocode is similar to algorithms but has a little more technical detail than an algorithm. It is still language-neutral. Below is a side-by-side comparison between an algorithm and pseudocode.
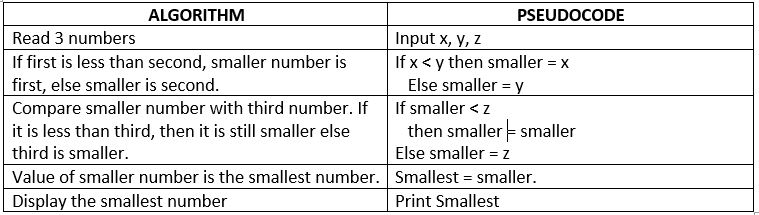
Use three numbers 5,3,4 and follow the Pseudocode steps above to get the smallest of the three.
If I wanted to add two numbers and divide the sum by 5, would the below Pseudocode work?
- Read x,y
- z=(x*y)/5
- PRINT z.
When the code does not give you the expected result, you will need to figure out where the error lies. An error in code is called a bug. Troubleshooting the error is called Debugging. Debugging is a key element of programming which we will look at in detail under Programming.
Pseudocode Summary
Pseudocode uses lot of code-style statements, but does not adhere to rules of any programming language. It helps with your analysis and design before you actually start coding.
Hands-on Activity
Write an algorithm in pseudocode that does a search for a letter in a word.